The Complete Python Pro Bootcamp
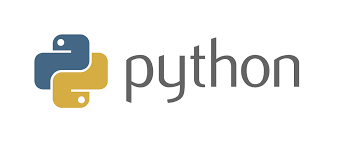
Python is a popular and versatile programming language known for its simplicity, readability, and extensive libraries. Here are some key concepts and features of Python:
-
Syntax:
- Python uses a clean and straightforward syntax, making it easy for beginners to learn and write code.
- Indentation (whitespace) is crucial for defining code blocks, such as loops and functions.
-
Data Types:
- Python supports various data types, including:
- Integers: Whole numbers (e.g., 5, -10).
- Floats: Decimal numbers (e.g., 3.14, -0.5).
- Strings: Text (e.g., “Hello, World!”).
- Booleans: True or False values.
- Lists: Ordered collections of items.
- Dictionaries: Key-value pairs.
- Tuples: Immutable sequences.
- Sets: Unordered collections of unique elements.
- Python supports various data types, including:
-
Variables:
- Variables store data values and can be assigned using the
=
operator. - Variable names are case-sensitive and follow certain rules (e.g., no spaces, start with a letter or underscore).
- Variables store data values and can be assigned using the
-
Control Flow:
- Conditional Statements:
if
,elif
, andelse
allow you to execute different code blocks based on conditions.
- Loops:
for
loops iterate over a sequence (e.g., a list).while
loops continue until a condition is met.
- Conditional Statements:
-
Functions:
- Functions are reusable blocks of code that perform specific tasks.
- You can define your own functions using the
def
keyword.
-
Libraries and Modules:
- Python has a vast ecosystem of libraries and modules for various purposes (e.g., data analysis, web development, machine learning).
- Popular libraries include NumPy, Pandas, Matplotlib, and Requests.
-
File Handling:
- Python can read and write files (e.g., text files, CSV files) using built-in functions.
-
Exception Handling:
- You can handle errors using
try
,except
, andfinally
blocks.
- You can handle errors using
-
Object-Oriented Programming (OOP):
- Python supports OOP principles like classes, objects, inheritance, and polymorphism.
-
Interactive Shell and Scripts:
- Python can be run interactively in a shell (REPL) or executed from script files (
.py
files).
- Python can be run interactively in a shell (REPL) or executed from script files (
Python Basics
Be the first to add a review.
Please, login to leave a review